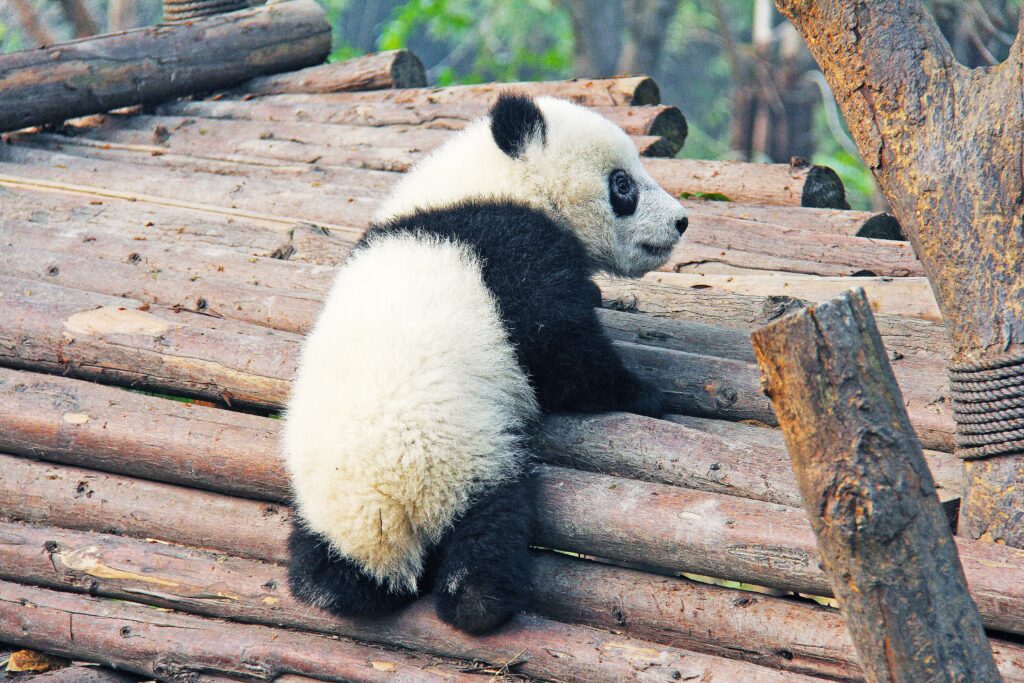
Welcome to the practical world of data manipulation with Python’s Pandas library! In the realm of data analysis, the ability to use the Pandas rename column feature in Pandas DataFrames is a key skill. It’s not just about changing names; it’s about bringing clarity and consistency to your data, crucial for effective analysis.
In this guide, we’ll explore various ways to use rename columns in Pandas. Join us as we delve into these methods, making your journey through Pandas both enlightening and practical.
Basics of Pandas DataFrame
Before we dive into the various methods to rename columns in Pandas, it’s essential to understand the foundation of Pandas – the DataFrame. A DataFrame is the central data structure in Pandas, used for storing and manipulating tabular data. Think of it as a table with rows and columns, much like a spreadsheet or SQL table.
First, import Pandas. If you haven’t installed Pandas yet, you can do so using pip:
pip install pandas
Now, let’s write a Python script to create a DataFrame:
import pandas as pd
# Sample data for creating a DataFrame
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
}
# Creating the DataFrame
df = pd.DataFrame(data)
# Display the DataFrame
print(df)
This code creates a DataFrame df
with three columns labeled ‘A’, ‘B’, and ‘C’, each containing three integer values. When you run this script, you’ll see a simple table output, forming the basis for our upcoming pandas rename column operations.
Understanding DataFrames is fundamental to mastering Pandas. As we proceed to the next sections, keep in mind that the operations we perform, especially renaming columns, are meant to enhance the readability and usability of these DataFrames in your data analysis projects.
Method 1 – Using the rename() Method
One of the most straightforward methods to use pandas to rename columns is using the rename()
method. This function in Pandas is specifically designed for altering index labels and column names. Let’s explore how you can use it for both single and multiple column renamings.
Explanation of Pandas
rename() Method
The rename()
method allows for flexibility in renaming. You can choose to rename just one column or multiple columns at once. Here’s the basic structure of the method:
DataFrame.rename(columns={'OldColumnName': 'NewColumnName'}, inplace=True)
columns
is a parameter where we pass a dictionary. The key represents the old column name, and the value is the new column name.inplace
is an optional parameter. If set toTrue
, it will modify the DataFrame in place, meaning the original DataFrame is changed. IfFalse
(default), it creates a new DataFrame with the changes.
Renaming Single and Multiple Columns in Pandas
Single Column:
# Renaming a single columndf2 = df.rename(columns={'A': 'Alpha'}, inplace=False)
This renames column ‘A’ to ‘Alpha’.
Multiple Columns:
# Renaming multiple columns
df2 = df.rename(columns={'A': 'Alpha', 'B': 'Beta'}, inplace=False)
Here, columns ‘A’ and ‘B’ are renamed to ‘Alpha’ and ‘Beta’, respectively.
Using inplace=True
If you want the changes to be applied directly to the original DataFrame, use inplace=True
:
# Modifying the DataFrame in place
df.rename(columns={'A': 'Alpha'}, inplace=True)
Example
Here’s a complete example:
import pandas as pd
# Sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
# Rename columns
df2 = df.rename(columns={'A': 'First', 'B': 'Second'}, inplace=False)
# Display the new DataFrame
print(df2)
Pros and Cons
Pros:
- Flexibility: Allows renaming of specific columns without affecting others.
- In-place Modification: Can modify the existing DataFrame without creating a new one, which is memory efficient.
Cons:
- Temporary Change: If not using
inplace=True
, you need to assign the result to a new DataFrame or overwrite the existing one. - Syntax Complexity: For beginners, understanding dictionary syntax for renaming can be a bit complex.
Method 2 – Changing the columns Attribute
Another effective method for renaming columns with Pandas is by directly modifying the columns
attribute of a DataFrame. This approach is particularly useful when you need to rename all columns at once or when you’re comfortable working with lists.
Modifying the columns Attribute in Pandas
Every Pandas DataFrame has an attribute named columns
that holds the names of its columns. By altering this attribute, you can efficiently rename columns.
Step-by-Step Guide
Renaming All Columns:
To rename all columns in a DataFrame, you simply assign a new list of column names to the columns
attribute.
df.columns = ['NewName1', 'NewName2', 'NewName3']
This code replaces all the existing column names with ‘NewName1’, ‘NewName2’, and ‘NewName3’. Ensure that the length of your new list matches the number of columns in the DataFrame.
Renaming Specific Columns While Keeping Others Intact:
If you only want to rename specific columns, you need to be a bit more careful. First, copy the existing column names into a list, modify the names you want to change, and then reassign this list back to df.columns
.
# Copying existing column names
new_columns = df.columns.tolist()
# Modifying specific columns
new_columns[0] = 'NewName1' # Changing the first column name
# Reassigning the modified list
df.columns = new_columns
Example
Here’s a full example:
import pandas as pd
# Sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
# Rename all columns
df.columns = ['First', 'Second', 'Third']
# Display the updated DataFrame
print(df)
Comparison with the rename() Method in Pandas
Advantages of Using columns
Attribute:
- Simplicity: This method is straightforward, especially when renaming all columns.
- Directness: It allows direct manipulation of the column names.
Disadvantages:
- Less Flexibility: It’s less flexible compared to the
rename()
method, particularly for renaming only a few columns. - Risk of Errors: There’s a risk of misaligning columns if the new list of names doesn’t match the original number of columns exactly.
By understanding how to modify the columns
attribute, you enhance your capabilities, giving you more control over your DataFrame’s structure. Next, we will look at another method, adding more depth to your Pandas expertise.
Using a Dictionary for Renaming Columns
When it comes to the “pandas rename column” task, using a dictionary is a powerful and flexible method. This approach is particularly useful when you need to rename multiple columns in a DataFrame, as it allows for clear mapping from old column names to new ones.
Utilizing a Dictionary for Renaming
In Pandas, a dictionary can be used to specify the changes you want to make in column names. This involves creating a dictionary where the keys are the current column names and the values are the new names you want to assign.
Step-by-Step Guide
Mapping Old Column Names to New Ones:
The first step is to create a dictionary that maps existing column names to their new names. For example:
rename_dict = {'OldName1': 'NewName1', 'OldName2': 'NewName2'}
Combining with rename()
Method:
Once you have your dictionary, you use it with the rename()
method to apply the changes to your DataFrame.
df.rename(columns=rename_dict, inplace=True)
Example
Here’s a complete example illustrating this method:
import pandas as pd
# Sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
# Dictionary for renaming columns
rename_dict = {'A': 'Alpha', 'B': 'Beta'}
# Rename columns using the dictionary
df.rename(columns=rename_dict, inplace=True)
# Display the updated DataFrame
print(df)
Situations Where This Method is Most Useful
- Selective Renaming: This method shines when you need to selectively rename a few columns without affecting others.
- Clarity in Code: Using a dictionary makes your intentions clear, especially when dealing with multiple column renamings.
- Batch Renaming: It’s useful in scenarios where you have a large DataFrame and need to rename several columns at once.
By incorporating dictionaries into your toolkit, you add another level of efficiency when renaming columns with pandas. This approach is especially handy for making specific, targeted changes to column names.
Advanced Tip – Renaming Columns While Reading Data in Pandas
An advanced and efficient approach to pandas renaming of columns is to rename columns during the initial data import stage. This can be particularly useful when working with large datasets or when you want to streamline your data processing workflow. Pandas provides this capability through functions like read_csv()
and others used for data import.
Renaming Columns During Data Import
When importing data using Pandas, you can rename columns directly within the read_csv()
function (or similar functions for different file formats) using the names
parameter. This approach is highly efficient, as it eliminates the need for a separate column renaming step after loading the data.
Example Code and Practical Scenarios
Here’s how you can rename columns while reading a CSV file:
import pandas as pd
# Specify new column names
new_column_names = ['Column1', 'Column2', 'Column3']
# Read the CSV file and rename columns
df = pd.read_csv('path/to/your/file.csv', names=new_column_names, header=0)
# Display the DataFrame
print(df)
In this example, names=new_column_names
specifies the new column names, and header=0
tells Pandas that the first row of the file contains the original column names, which should be replaced.
Practical Scenarios
- Large Datasets: When dealing with large datasets, renaming columns during import can save processing time and memory.
- Standardizing Column Names: If you regularly work with datasets that have similar structures but inconsistent column names, this method allows you to standardize column names across different files easily.
- Streamlining Data Processing Pipelines: By renaming columns at the import stage, you can create more streamlined and efficient data processing pipelines.
Renaming columns while reading data into a Pandas DataFrame is a powerful technique that can significantly enhance your data handling capabilities. It’s a smart move for “pandas rename column” tasks, especially in scenarios where efficiency and consistency are key.
Best Practices and Common Pitfalls for renaming a column in Pandas
As you become more familiar with the processes for renaming pandas columns, it’s essential to be aware of best practices and common pitfalls. This knowledge will help you rename columns more effectively and avoid common errors.
Best Practices for Renaming Columns
- Consistent Naming Conventions: Use consistent naming conventions across your datasets. This includes deciding on using either CamelCase, snake_case, or another style and sticking to it.
- Descriptive Names: Choose column names that are descriptive and convey the meaning of the data they hold. This makes your data more readable and understandable.
- Check for Typos: Always double-check for typos in your new column names. A small typo can lead to errors or confusion later in your data analysis.
- Use
inplace
Judiciously: Be cautious when usinginplace=True
in therename()
method. While it saves memory by modifying the DataFrame in place, it also means you lose the original DataFrame structure. - Backup Original Data: Before renaming columns, especially in large datasets, it’s a good idea to keep a backup of the original DataFrame. This allows you to revert to the original state in case something goes wrong.
Common Pitfalls to Avoid
- Mismatch in Column Names Length: When using the method of directly modifying the
columns
attribute, ensure the length of your new list matches the number of columns in the DataFrame. A mismatch can lead to errors or misaligned data. - Overwriting Columns Unintentionally: Be careful not to assign the same name to multiple columns, as this can lead to overwriting data.
- Ignoring Case Sensitivity: Remember that column names are case-sensitive. ‘Column’ and ‘column’ are considered different.
- Forgetting to Assign the Result: When using the
rename()
method withoutinplace=True
, remember to assign the result to a DataFrame, either new or existing. - Not Verifying After Renaming: Always verify the DataFrame after renaming columns to ensure the changes have been applied correctly.
By following these best practices and being aware of common pitfalls, you can make the “pandas rename column” process smoother and more error-free. This not only improves your data manipulation skills but also ensures the integrity and quality of your data analysis.
Conclusion
We’ve explored a range of methods, each offering its unique approach and advantages for renaming columns in DataFrames. From the versatile rename()
method to directly manipulating the columns
attribute, and even employing dictionaries for precise renaming, you’ve now got a toolkit brimming with options.
Remember, the key to mastering Pandas, particularly the nuances of “python pandas rename column” processes, is practice. Each method we’ve discussed has its place, and becoming comfortable with each will significantly enhance your data analysis skills. Experiment with different datasets, try renaming columns in various ways, and see firsthand how these techniques can streamline your data manipulation tasks.
For further reading and to solidify your understanding, I highly recommend visiting the official Pandas documentation. It’s an invaluable resource for deepening your knowledge and staying updated with the latest Pandas features.
If you’re eager to expand your Pandas expertise, consider exploring practicing and learning on kaggle.
As you continue your journey in Python’s world of data science, remember that learning is a continuous process. The more you practice and explore, the more skilled you’ll become. So, keep experimenting, keep learning, and let Pandas be your trusted companion in all your data endeavors!